Bootstrap & Webpack
Gid ofisyèl la pou konnen kijan pou mete ak pake CSS Bootstrap ak JavaScript nan pwojè w la lè l sèvi avèk Webpack.
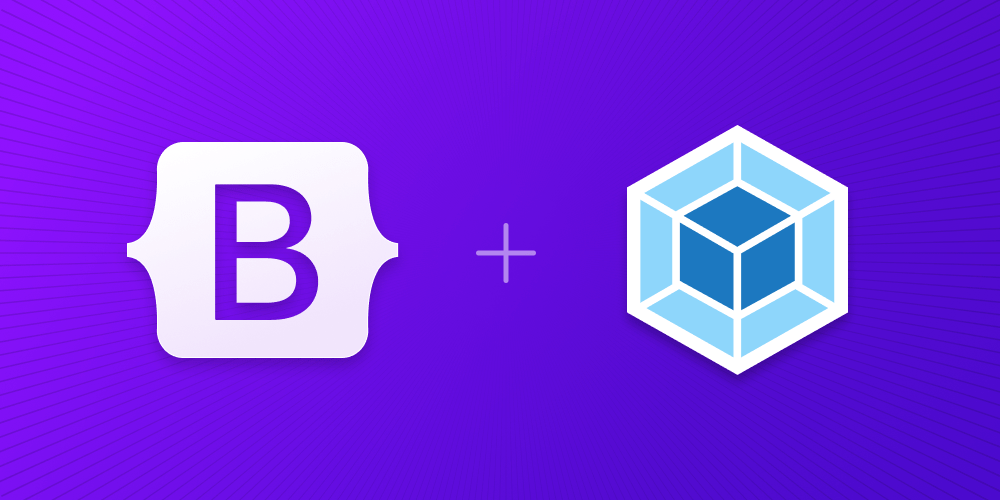
Enstalasyon
Nou ap bati yon pwojè Webpack ak Bootstrap depi nan grafouyen, kidonk gen kèk kondisyon ak etap anvan nou ka reyèlman kòmanse. Gid sa a mande pou ou gen Node.js enstale ak kèk abitye ak tèminal la.
-
Kreye yon katab pwojè ak konfigirasyon npm. Nou pral kreye
my-project
katab la epi inisyalize npm ak-y
agiman an pou evite li mande nou tout kesyon entèaktif yo.mkdir my-project && cd my-project npm init -y
-
Enstale Webpack. Apre sa, nou bezwen enstale depandans devlopman Webpack nou an:
webpack
pou nwayo Webpack,webpack-cli
pou nou ka kouri kòmandman Webpack soti nan tèminal la, epiwebpack-dev-server
pou nou ka kouri yon sèvè devlopman lokal. Nou itilize--save-dev
pou siyal ke depandans sa yo se sèlman pou itilizasyon devlopman epi yo pa pou pwodiksyon.npm i --save-dev webpack webpack-cli webpack-dev-server
-
Enstale Bootstrap. Koulye a, nou ka enstale Bootstrap. Nou pral enstale Popper tou paske dropdowns, popovers, ak konsèy sou zouti nou yo depann sou li pou pwezante yo. Si ou pa planifye sou itilize eleman sa yo, ou ka omisyon Popper isit la.
npm i --save bootstrap @popperjs/core
-
Enstale depandans adisyonèl. Anplis Webpack ak Bootstrap, nou bezwen kèk plis depandans pou byen enpòte ak pake Bootstrap a CSS ak JS ak Webpack. Men sa yo enkli Sass, kèk chajè, ak Autoprefixer.
npm i --save-dev autoprefixer css-loader postcss-loader sass sass-loader style-loader
Kounye a ke nou gen tout depandans ki nesesè yo enstale, nou ka jwenn nan travay kreye dosye yo pwojè ak enpòte Bootstrap.
Estrikti pwojè
Nou te deja kreye my-project
katab la ak inisyalize npm. Koulye a, nou pral tou kreye nou yo src
ak dist
dosye yo awondi estrikti pwojè a. Kouri sa ki annapre yo soti nan my-project
, oswa manyèlman kreye katab ak estrikti dosye yo montre anba a.
mkdir {dist,src,src/js,src/scss}
touch dist/index.html src/js/main.js src/scss/styles.scss webpack.config.js
Lè w fini, pwojè konplè ou a ta dwe sanble sa a:
my-project/
├── dist/
│ └── index.html
├── src/
│ ├── js/
│ │ └── main.js
│ └── scss/
│ └── styles.scss
├── package-lock.json
├── package.json
└── webpack.config.js
Nan pwen sa a, tout bagay an bon plas, men Webpack pa pral travay paske nou poko ranpli nan nou an webpack.config.js
.
Konfigure Webpack
Avèk depandans enstale ak katab pwojè nou an pare pou nou kòmanse kodaj, nou ka kounye a konfigirasyon Webpack epi kouri pwojè nou an lokalman.
-
Louvri
webpack.config.js
nan editè ou. Depi li vid, nou pral bezwen ajoute kèk konfigirasyon boilerplate nan li pou nou ka kòmanse sèvè nou an. Pati sa a nan konfigirasyon an di Webpack yo te chèche JavaScript pwojè nou an, ki kote yo soti kòd la konpile a (dist
), ak ki jan sèvè devlopman an ta dwe konpòte (rale soti nandist
katab la ak rechaje cho).const path = require('path') module.exports = { entry: './src/js/main.js', output: { filename: 'main.js', path: path.resolve(__dirname, 'dist') }, devServer: { static: path.resolve(__dirname, 'dist'), port: 8080, hot: true } }
-
Apre sa nou ranpli
dist/index.html
. Sa a se paj HTML Webpack la pral chaje nan navigatè a pou itilize CSS ak JS ki pakè nou pral ajoute sou li nan etap pita yo. Anvan nou ka fè sa, nou dwe bay li yon bagay pou rann epi enklioutput
JS ki soti nan etap anvan an.<!doctype html> <html lang="en"> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <title>Bootstrap w/ Webpack</title> </head> <body> <div class="container py-4 px-3 mx-auto"> <h1>Hello, Bootstrap and Webpack!</h1> <button class="btn btn-primary">Primary button</button> </div> <script src="./main.js"></script> </body> </html>
Nou ap enkli yon ti kras nan stil Bootstrap isit la ak la
div class="container"
ak<button>
pou nou wè lè CSS Bootstrap a chaje pa Webpack. -
Koulye a, nou bezwen yon script npm pou kouri Webpack. Louvri
package.json
epi ajoutestart
script ki montre anba a (ou ta dwe deja gen script tès la). Nou pral sèvi ak script sa a pou kòmanse sèvè lokal Webpack dev nou an.{ // ... "scripts": { "start": "webpack serve --mode development", "test": "echo \"Error: no test specified\" && exit 1" }, // ... }
-
Epi finalman, nou ka kòmanse Webpack. Soti nan
my-project
katab la nan tèminal ou a, kouri sa a ki fèk ajoute script npm:npm start
Nan pwochen ak dènye seksyon gid sa a, nou pral mete kanpe chajè Webpack yo epi enpòte tout CSS ak JavaScript Bootstrap la.
Enpòte Bootstrap
Enpòte Bootstrap nan Webpack mande pou chajè yo nou enstale nan premye seksyon an. Nou te enstale yo ak npm, men kounye a Webpack bezwen konfigirasyon pou itilize yo.
-
Mete chajè yo nan
webpack.config.js
. Fichye konfigirasyon ou an konplè kounye a epi li ta dwe matche ak ti bout ki anba a. Sèl pati nan nouvo isit la semodule
seksyon an.const path = require('path') module.exports = { entry: './src/js/main.js', output: { filename: 'main.js', path: path.resolve(__dirname, 'dist') }, devServer: { static: path.resolve(__dirname, 'dist'), port: 8080, hot: true }, module: { rules: [ { test: /\.(scss)$/, use: [ { loader: 'style-loader' }, { loader: 'css-loader' }, { loader: 'postcss-loader', options: { postcssOptions: { plugins: () => [ require('autoprefixer') ] } } }, { loader: 'sass-loader' } ] } ] } }
Men yon rezime poukisa nou bezwen tout chajè sa yo.
style-loader
enjekte CSS la nan yon<style>
eleman nan<head>
paj HTML la,css-loader
ede w itilize@import
akurl()
,postcss-loader
li nesesè pou Autoprefixer, epi lisass-loader
pèmèt nou sèvi ak Sass. -
Koulye a, an n enpòte CSS Bootstrap la. Ajoute sa ki annapre yo
src/scss/styles.scss
pou enpòte tout sous Bootstrap Sass.// Import all of Bootstrap's CSS @import "~bootstrap/scss/bootstrap";
Ou kapab tou enpòte stylesheets nou yo endividyèlman si ou vle. Li dokiman Sass enpòte nou an pou plis detay.
-
Apre sa, nou chaje CSS la epi enpòte JavaScript Bootstrap la. Ajoute sa ki annapre yo
src/js/main.js
pou chaje CSS la epi enpòte tout JS Bootstrap la. Popper pral enpòte otomatikman atravè Bootstrap.// Import our custom CSS import '../scss/styles.scss' // Import all of Bootstrap's JS import * as bootstrap from 'bootstrap'
Ou ka enpòte grefon JavaScript tou endividyèlman jan sa nesesè pou kenbe gwosè pake yo:
import Alert from 'bootstrap/js/dist/alert' // or, specify which plugins you need: import { Tooltip, Toast, Popover } from 'bootstrap'
Li dokiman JavaScript nou yo pou plis enfòmasyon sou kijan pou itilize grefon Bootstrap yo.
-
Epi ou fini! 🎉 Avèk sous Bootstrap Sass ak JS konplètman chaje, sèvè devlopman lokal ou a ta dwe kounye a sanble tankou sa a.
Koulye a, ou ka kòmanse ajoute nenpòt eleman Bootstrap ou vle itilize. Asire w ke w tcheke pwojè egzanp Webpack konplè a pou konnen kijan pou w enkli plis Sass koutim epi optimize konstriksyon w lè w enpòte sèlman pati CSS ak JS Bootstrap ou bezwen yo.
Optimize pwodiksyon an
Tou depan de konfigirasyon ou a, ou ka vle aplike kèk sekirite adisyonèl ak optimize vitès ki itil pou kouri pwojè a nan pwodiksyon an. Remake byen ke optimize sa yo pa aplike sou pwojè egzanp Webpack la epi se ou menm pou w aplike.
Extracting CSS
The style-loader
we configured above conveniently emits CSS into the bundle so that manually loading a CSS file in dist/index.html
isn’t necessary. This approach may not work with a strict Content Security Policy, however, and it may become a bottleneck in your application due to the large bundle size.
To separate the CSS so that we can load it directly from dist/index.html
, use the mini-css-extract-loader
Webpack plugin.
First, install the plugin:
npm install --save-dev mini-css-extract-plugin
Then instantiate and use the plugin in the Webpack configuration:
--- a/webpack/webpack.config.js
+++ b/webpack/webpack.config.js
@@ -1,8 +1,10 @@
+const miniCssExtractPlugin = require('mini-css-extract-plugin')
const path = require('path')
module.exports = {
mode: 'development',
entry: './src/js/main.js',
+ plugins: [new miniCssExtractPlugin()],
output: {
filename: "main.js",
path: path.resolve(__dirname, "dist"),
@@ -18,8 +20,8 @@ module.exports = {
test: /\.(scss)$/,
use: [
{
- // Adds CSS to the DOM by injecting a `<style>` tag
- loader: 'style-loader'
+ // Extracts CSS for each JS file that includes CSS
+ loader: miniCssExtractPlugin.loader
},
{
After running npm run build
again, there will be a new file dist/main.css
, which will contain all of the CSS imported by src/js/main.js
. If you view dist/index.html
in your browser now, the style will be missing, as it is now in dist/main.css
. You can include the generated CSS in dist/index.html
like this:
--- a/webpack/dist/index.html
+++ b/webpack/dist/index.html
@@ -3,6 +3,7 @@
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
+ <link rel="stylesheet" href="./main.css">
<title>Bootstrap w/ Webpack</title>
</head>
<body>
Extracting SVG files
Bootstrap’s CSS includes multiple references to SVG files via inline data:
URIs. If you define a Content Security Policy for your project that blocks data:
URIs for images, then these SVG files will not load. You can get around this problem by extracting the inline SVG files using Webpack’s asset modules feature.
Configure Webpack to extract inline SVG files like this:
--- a/webpack/webpack.config.js
+++ b/webpack/webpack.config.js
@@ -16,6 +16,14 @@ module.exports = {
},
module: {
rules: [
+ {
+ mimetype: 'image/svg+xml',
+ scheme: 'data',
+ type: 'asset/resource',
+ generator: {
+ filename: 'icons/[hash].svg'
+ }
+ },
{
test: /\.(scss)$/,
use: [
After running npm run build
again, you’ll find the SVG files extracted into dist/icons
and properly referenced from CSS.
See something wrong or out of date here? Please open an issue on GitHub. Need help troubleshooting? Search or start a discussion on GitHub.